Your cart is currently empty!

Grow Your Business with More Positive Google Reviews
We help businesses like yours increase trust, visibility, and credibility through real, organic Google reviews – all managed by our smart system.
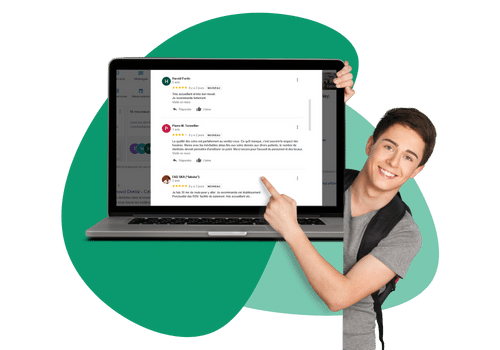
- 📈 Improved Google Maps and search visibility
- ⭐ Authentic and consistent customer reviews
- 🤖 Semi-automated review collection process
- 📬 Monthly performance summaries
Simple Plans, Powerful Value
” Boost your brand’s credibility with authentic Google reviews – tailored for every business size.”
$19.99
/month
🛠️ Starter Package
Ideal for freelancers, startups, and small service providers.
$49.99
/month
🚀Growth Package
Perfect for restaurants, clinics, local shops, and growing businesses.
$89.99
/month
👑Premium Package
Expert suggestions for continuous improvement

Turning Reviews Into Revenue
Investing in online reviews is not just about reputation — it’s a smart business move. Positive reviews build trust, attract more customers, and ultimately increase sales. By actively managing and improving your Google Reviews, you’re not just enhancing your brand image — you’re investing in long-term profit.

The Power of Trust: Why Reviews Matte
Customer reviews are one of the most powerful tools in digital marketing. They provide social proof, increase trust, and influence buying decisions. Businesses with strong, positive reviews stand out from the competition and appear more credible. Investing in your online reputation builds long-term trust that turns visitors into loyal customers.
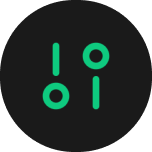
Review Templates
Choose from proven templates to ask for reviews in a friendly and professional tone

Real-Time Alerts
Get instantly notified when someone leaves a new Google review for your business

Smart AI Responses
Use AI-powered suggestions to respond quickly and smartly to all reviews
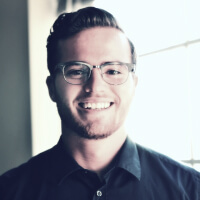
Liam Parker
Restaurant Owner
ReviewBoost changed the game for us. We used to get 1 or 2 reviews a month, now we get them weekly! Super easy to use too


Nina Rodriguez – Beauty Salon
Beauty Salon
Love this platform! Clients finally started leaving reviews without us begging them lol. It really helped us grow online

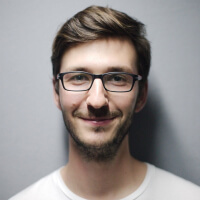
Mohamed El-Sayed
Dental Clinic
Great service. Professional setup, and now our Google rating went up from 4.1 to 4.7 in two months. Thank you ReviewBoost


Aiko Tanaka – Nail Studio
Nail Studio

I was worried about asking people for reviews, but ReviewBoost made it smooth and natural. Highly recommend it
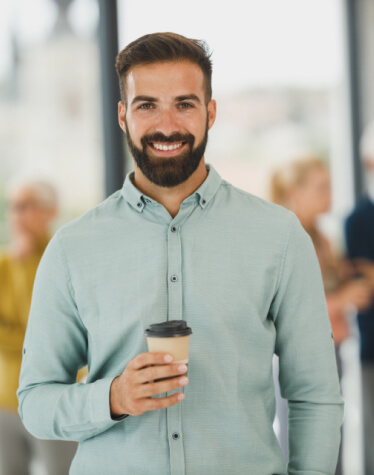
David Moreau
Fitness Studio
Our clients now actually leave feedback, and it’s all because of ReviewBoost. Super happy with the results

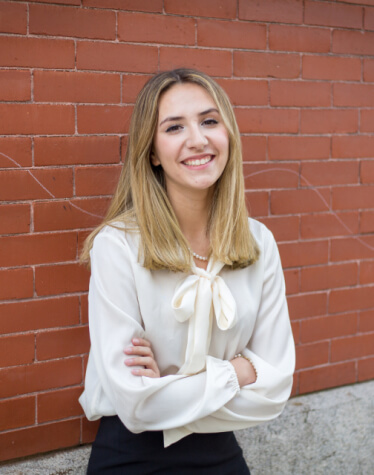
Fatima Khan
Small Business Owner
Didn’t know how important Google reviews were until I tried this platform. It made everything so simple. Big thanks
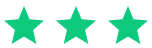
Get Started
Elevate Your Business with Smart, Scalable Solutions!
Lorem ipsum is placeholder text commonly used in the graphic, print, and publishing industries for previewing layouts and visual mockups.